DIY Arduino Oscilloscope with the Nokia 3310 GLCD screen
I’ve seen videos on YouTube using an Arduino and a Graphical LCD
screen (GLCD) to create a simple Oscilloscope. The annoying thing is
that I found no help whatsoever on how to build one. I spent a few days
figuring out how to use the Nokia 3310 LCD screen and then figuring out
how to sample an analog port to create a fun oscilloscope effect.
I don’t claim that this device can replace actual test equipment but
it might be useful and for $40.00 bucks in total parts it is a blast to
play with.
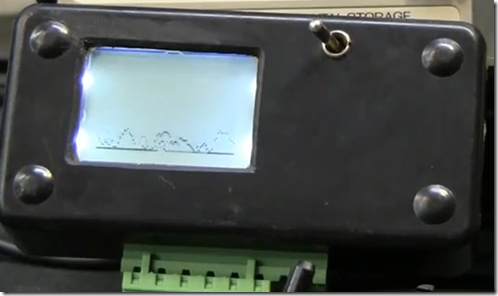
The setup is really simple, connect an Arduino Pro Mini to a Nokia
3310 LCD screen, sample an analog port and then wright the pixels to the
screen. I even added two potentiometers, one that adds delay to the
sample to essentially provide a basic time scale and another pot that
can scale down voltages as long as they are below three volts (the
operating voltage of the micro controller). Unfortunately if you want to
sample sign waves that have voltages over three volts you are out of
luck unless you build an electronic circuit to scale it down somehow.
Another unfortunate feature is that the analog input only shows
positive voltage so the scope is really a positive DC or AC scope only.
Here again custom electronics could be built but there is something
beautiful about keeping it simple.
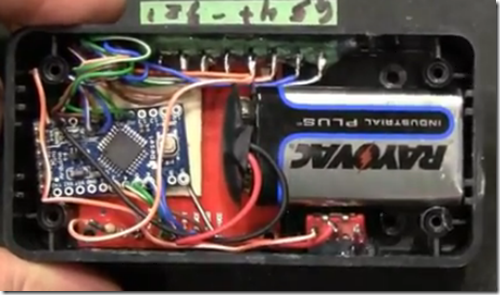
I don’t have a professional function generator so I could only test its range from 100 ms to about 1 ms scales.
Bottom line, it is definitely fun to build and play with, it also makes a cool EMF detector
Supporting files:
ArduinoOscilloscope.zip">Arduino Oscilloscope.zip
Schematic: Arduino Oscilloscope.pdf
Here is the Sketch
02 | ########################################################### |
03 | Title: Arduino Oscilloscope |
04 | Purpose: Use a Nokia 3310 GLCD screen with the arduino |
05 | Created by: Fileark. see Fileark.com for more info. |
06 | Note: Please reuse, repurpose, and redistribute this code. |
07 | Note: This code uses the Adafruit PDC8544 LCD library |
08 | ########################################################### |
13 | // pin 3 - Serial clock out (SCLK) |
14 | // pin 4 - Serial data out (DIN) |
15 | // pin 5 - Data/Command select (D/C) |
16 | // pin 7 - LCD chip select (CS) |
17 | // pin 6 - LCD reset (RST) |
18 | PCD8544 nokia = PCD8544(3, 4, 5, 7, 6); |
20 | // a bitmap of a 16x16 fruit icon |
21 | static unsigned char __attribute__ ((progmem)) logo16_glcd_bmp[]={ |
22 | 0x06, 0x0D, 0x29, 0x22, 0x66, 0x24, 0x00, 0x01, 0x87, 0x00, 0x27, 0x6C, 0x20, 0x23, 0x06, 0x00, |
23 | 0x00, 0x00, 0x00, 0x00, 0x00, 0x10, 0x10, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, }; |
24 | #define LOGO16_GLCD_HEIGHT 16 |
25 | #define LOGO16_GLCD_WIDTH 16 |
27 | int channelAI = A0; // select the input pin for the Oscilioscope |
28 | int scaleYAI = A1; // select the input pin for the Y (horizontal) potentiometer |
29 | int scaleXAI = A2; // select the input pin for the X (Vertical) potentiometer |
31 | int delayVariable = 0; // define a variable for the Y scale / delay |
32 | int xVariable = 0; // define a variable for the x scale |
33 | int
yCtr =
0; //
define a variable for the y counter used to collect y position into
array |
34 | int
posy =
0; //
define a variable for the y position of the dot |
35 | int myArray[85]; // define an array to hold the data coming in |
43 | // turn all the pixels on (a handy test) |
44 | nokia.command(PCD8544_DISPLAYCONTROL | PCD8544_DISPLAYALLON); |
47 | nokia.command(PCD8544_DISPLAYCONTROL | PCD8544_DISPLAYNORMAL); |
57 | delayVariable = analogRead(scaleYAI); |
58 | delayVariable = (delayVariable/50); |
59 | xVariable = analogRead(scaleXAI); |
60 | xVariable = (xVariable/22); |
62 | for(yCtr = 0; yCtr < 85; yCtr += 1) // the for loop runs from 0 and < 85, it fills the array with 84 records |
64 | posy = analogRead(channelAI); // read the value from the sensor: |
65 | myArray[yCtr]
= (posy/xVariable); // scale the value based on the x scale
potentiometer |
66 | delay
(delayVariable);
// scale the y collection of data using the delay from the y
potentiometer |
69 | yCtr
==
0;
// set the counter to zero so we can use it again |
70 | nokia.clear();
// clear the LCD screen so we can draw new pixels |
72 | for(yCtr = 0; yCtr < 85; yCtr += 1) // for loop runs 84 times |
74 | nokia.setPixel(yCtr, myArray[yCtr], BLACK); // draw the 84 pixels on the screen |
77 | nokia.display();
// show the changes to the buffer |
78 | yCtr
==
0;
// set the counter to zero so we can use it again |